video
A Youtube or Vimeo video embed
Preview
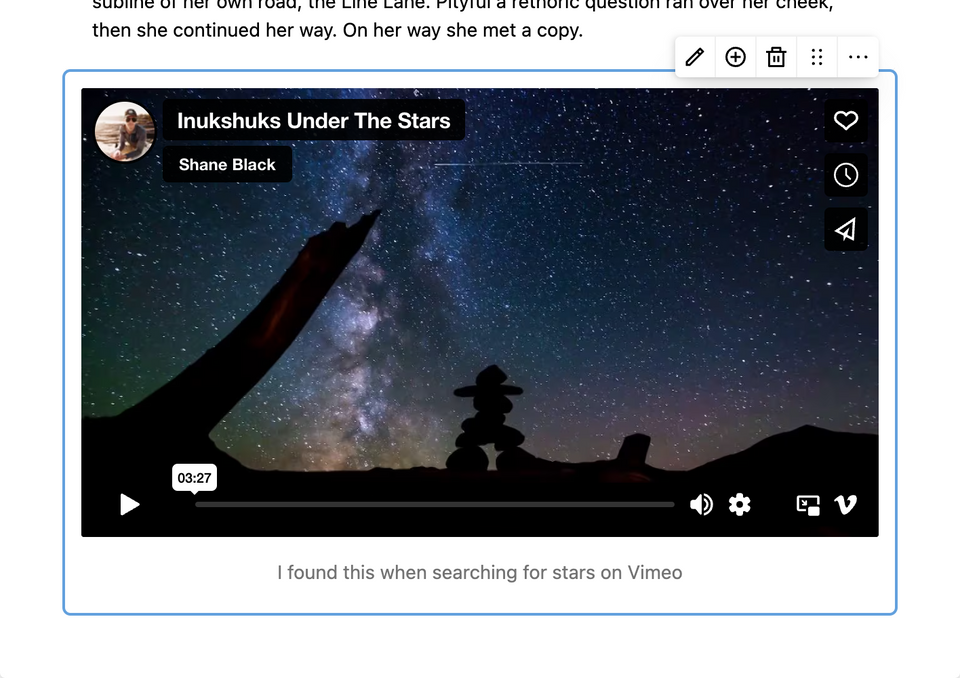
Default files
Snippet
<?php
use Kirby\Cms\Html;
/** @var \Kirby\Cms\Block $block */
$caption = $block->caption();
if (
$block->location() == 'kirby' &&
$video = $block->video()->toFile()
) {
$url = $video->url();
$attrs = array_filter([
'autoplay' => $block->autoplay()->toBool(),
'controls' => $block->controls()->toBool(),
'loop' => $block->loop()->toBool(),
'muted' => $block->muted()->toBool() || $block->autoplay()->toBool(),
'playsinline' => $block->autoplay()->toBool(),
'poster' => $block->poster()->toFile()?->url(),
'preload' => $block->preload()->value(),
]);
} else {
$url = $block->url();
}
?>
<?php if ($video = Html::video($url, [], $attrs ?? [])): ?>
<figure>
<?= $video ?>
<?php if ($caption->isNotEmpty()): ?>
<figcaption><?= $caption ?></figcaption>
<?php endif ?>
</figure>
<?php endif ?>
To overwrite this default snippet, place your custom file in /site/snippets/blocks/video.php
.
Blueprint
name: field.blocks.video.name
icon: video
preview: video
fields:
location:
label: field.blocks.video.location
type: radio
columns: 2
default: "web"
options:
kirby: "{{ t('field.blocks.image.location.internal') }}"
web: "{{ t('field.blocks.image.location.external') }}"
url:
label: field.blocks.video.url.label
type: url
placeholder: field.blocks.video.url.placeholder
when:
location: web
video:
label: field.blocks.video.name
type: files
query: model.videos
multiple: false
# you might want to add a template for videos here
when:
location: kirby
poster:
label: field.blocks.video.poster
type: files
query: model.images
multiple: false
image:
back: black
uploads:
template: blocks/image
when:
location: kirby
caption:
label: field.blocks.video.caption
type: writer
inline: true
autoplay:
label: field.blocks.video.autoplay
type: toggle
width: 1/3
when:
location: kirby
muted:
label: field.blocks.video.muted
type: toggle
width: 1/3
default: true
when:
location: kirby
loop:
label: field.blocks.video.loop
type: toggle
width: 1/3
when:
location: kirby
controls:
label: field.blocks.video.controls
type: toggle
width: 1/3
default: true
when:
location: kirby
preload:
label: field.blocks.video.preload
type: select
width: 2/3
default: auto
options:
- auto
- metadata
- none
when:
location: kirby
To overwrite this default blueprint, place your custom file in /site/blueprints/blocks/video.yml
.
Vue component
kirby/blob/main/panel/src/components/Forms/Blocks/Types/Video.vue